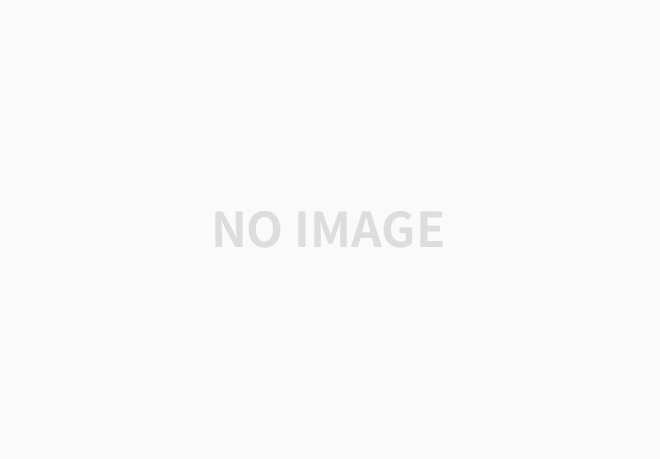
const contentAPI = [
{
tab: "section 1",
content: "i am the content of the section1"
},
{
tab: "section 2",
content: "i am the content of the section2"
}
];
const useTab = (initialTab, allTabs) => {
// allTabs 이 배열이 아닐 때 함수 종료
if (!allTabs || !Array.isArray(allTabs)) {
return;
}
const [currentIndex, setCurrentIndex] = useState(initialTab);
return {
currentItem: allTabs[currentIndex],
changeItem: setCurrentIndex
};
};
const App = () => {
// currentItem 은 useTab 커스텀 훅에 의하면
// allTabs의 currentIndex번째 아이템이다
const { currentItem, changeItem } = useTab(0, contentAPI);
return (
<div className="App">
<h1>Hello CodeSandbox </h1>
<div>
{contentAPI.map((section, index) => (
// changeItem(index) 을 onClick에 그대로 받으면
// 함수가 즉시 실행되기 때문에
// 익명 함수로 changeItem(index)를 전달한다
<button onClick={() => changeItem(index)}>{section.tab}</button>
))}
{/* contentAPI 배열의 currentItem 번째의 content 내용이 찍힌다 */}
<div>{currentItem.content}</div>
</div>
</div>
);
};
버튼 인덱스에 맞는 컨텐츠를 가져오는 로직은
1. onClick={() => changeItem(index)}
버튼 온클릭시 익명함수로 changeItem 을 커스텀훅인 useTab에 전달한다
2. changeItem: setCurrentIndex
changeItem 은 곧 setCurrentIndex 가 되고 useState에 의해
currentIndex 값이 갱신이 된다.
3. currentItem: allTabs[currentIndex]
currentItem이 리턴되어 컴포넌트에 {currentItem.content} 이 부분 역시 바뀐다.
'배운 것 > react & reacthooks' 카테고리의 다른 글
훅스에서 setState는 비동기다 (0) | 2019.12.25 |
---|---|
리액트 커스텀 훅 사용하기 => useInput (0) | 2019.12.21 |
리액트 훅 (Reack Hooks) 간단 소개 (0) | 2019.12.21 |
[react] axios, yts api (0) | 2019.10.24 |
[react] state, componentDidMount (0) | 2019.10.23 |